As soon as it appeared, React immediately changed the rules of the game. It ushered in a new era in front-end development. Its unidirectional data flow, JSX, and declarative way of defining views was just crazy. React quickly gained immense popularity, and even today, 6 years later, it remains one of the most popular front-end libraries.
However, the frontend ecosystem is growing rapidly, and a lot of interesting projects are being developed in this area. We are constantly on the lookout for the next technology that will once again be a game-changer. We like it when yesterday’s outsider goes aces, completely undermining the way we work – as React did 6 years ago.
The joy of finding new technology to help you be more productive than ever before is priceless. It is very important to study what else besides React exists around, even if you consider yourself to be a React specialist. A good developer chooses the tools he needs to solve the problem himself. He needs to know many paradigms, languages and tools.
We have done some research lately, experimented and found some really interesting libraries and new web standards that we think have potential. Everything we’ll cover in this post is available for use in production today. These technologies cannot be called “cutting edge”, but they have not yet become widespread.
Svelte
Let’s start with brand new frameworks that instill new ways of thinking rather than reimagining something that already exists. This is Svelte. This is a new paradigm in the customer ecosystem.
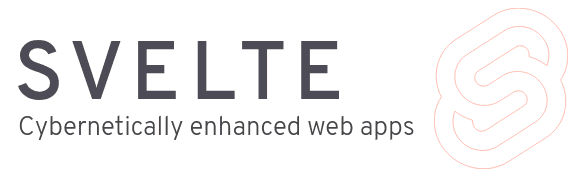
So why is Svelte unique? Mainly for two reasons. First, it creates insanely small packages because it doesn’t include the runtime. Svelte advocates call this the “zero runtime”. The React runtime weighs in at about 100kb, and this space is added on top of the package for each React application.
The second major strength of Svelte is its syntax. It’s so minimal, as if the syntax isn’t there at all. We will return to this later.
Svelte borrows a lot from React and Vue… If you are experienced with React, then you already know a lot, and you will hardly need time to master Svelte.
Let’s take a look at some code to get an impression of Svelte.
To define a new component, a new file is created and the name of this file will be the name of the component. This file will have an optional script section, an optional styles section and, importantly, the HTML template itself. Svelte does not use JSX, but uses its own templating language.
<script>
export let counter;
</script>
<style>
p {
color: purple;
}
</style>
<p>The counter is {counter}</p>
A bit like Vue, but simpler.
Syntax … and there is no special syntax, it’s just pure JavaScript. Want to set a state? Just increase the variable counter
.
<script>
let count = 0;
function handleClick() {
count += 1;
}
</script>
<button on:click={handleClick}>
Clicked {count} {count === 1 ? 'time' : 'times'}
</button>
No this.setState
. No hooks useState
. Just JavaScript. With such small examples, it is difficult to appreciate the entire minimalism of the syntax. We programmed like this for several hours, and it turned out super productive, since we had to write so much less code to solve the problem. It seemed to me as if we were running, and someone else was pushing us in the back. This minimalistic syntax is a deliberate decision made by the creators of Svelte. They explain themselves in the Write less code post why.
The most important part of a web framework is its documentation. React is famous for its great documentation, many people learn it only from the docks. Svelte also has really good documentation as far as we can tell. As a beginner to Svelte, we like the examples in the documentation for this framework the most : In many cases, we know roughly what we need. For example, change the state of a component, add event handlers, get some global state (like in Redux). Next, we need to quickly see how it’s done. The examples section is an example of this kind. We don’t have to google articles on any blogs or look at listings on Github. Just browse the section you want. Beauty!
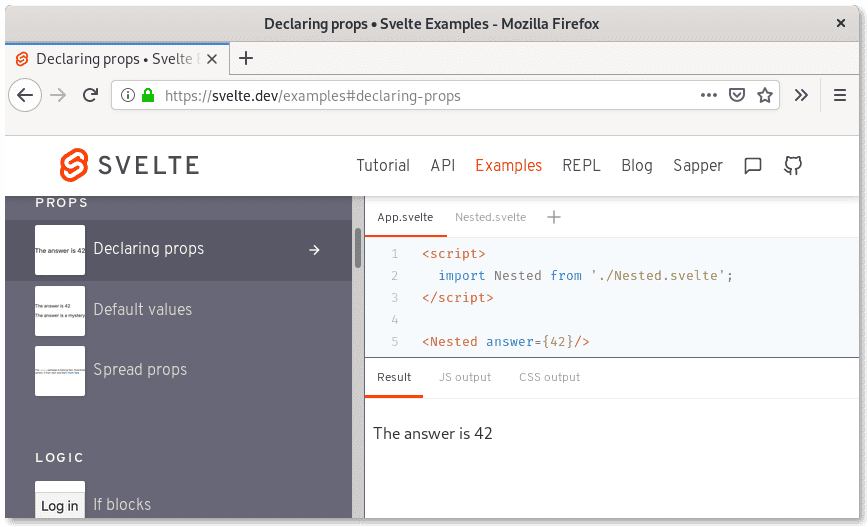
There is even something similar to Gatsby and Next.js. The thing is called Sapper , you start coding very quickly. Allows you to create single page applications with static generated HTML pages, which is similar to Gatsby / Next.js.
The Svelte Redux also has an alternative, called stores. They are incredibly minimalistic. The repository is created like this:
import { writable } from 'svelte/store';
export const count = writable(0);
Then the repository can be updated like this.
count.set(0);
There is no stereotyped code at all, and this whole feature is entirely built into Svelte – no additional dependencies. Now, remember the hassle of adding Redux to your React project.
How does the React team feel about Svelte?
The React team isn’t as enthusiastic about Svelte as we are. They find that the performance gains associated with moving away from the runtime environment are negligible. Only in very specific cases, such as when working with inline widgets, etc. such a game is worth the candle. In addition, we have Gatsby and Next, so, according to the React community, a small increase in speed means even less. In this case, we argue that any improvement in speed matters, and dismissing the 100kb savings on each package just because “it only comes in handy with widgets” is bad argument. To make the web great and accessible, we have to do every kind of performance optimization we can. If you’ve ever tweaked an application to improve its performance, you know how much even the smallest improvements mean. It’s the sum of all the small improvements that add up to a super-fast download that happens like magic.
So, should I try Svelte?
Next time, when we start a project that would work well with React, we will seriously consider using Svelte instead. While researching Svelte, we were surprised how productive this framework is, even without reading the documentation. All we did – worked through the examples and practiced a bit with Sapper – and still felt like it was getting more productive than with React. We think this high productivity has something to do with the minimalist syntax. It is also very valuable that, without any additional work, we get such compact packages. Apps that load quickly are loved by both our users and Google!
Does Svelte have any disadvantages? To be honest, only one comes to my mind. The point is that Svelte is still new and not very widely used. Therefore, its community is limited, and, perhaps even more importantly, there are not many (yet?) Companies that need specialists in Svelte. If you’re a junior developer looking for a job, then we think it makes more sense for you to learn React first. However, if you are a senior developer (or a junior and you have a lot of time), we strongly recommend taking a closer look at Svelte.
Web components
Could web components be the next great technology? We think she definitely has potential. So what are web components?
Web Components is a set of w3c standards for creating new custom HTML tags. With these standards, you can create anything from widgets to large applications.
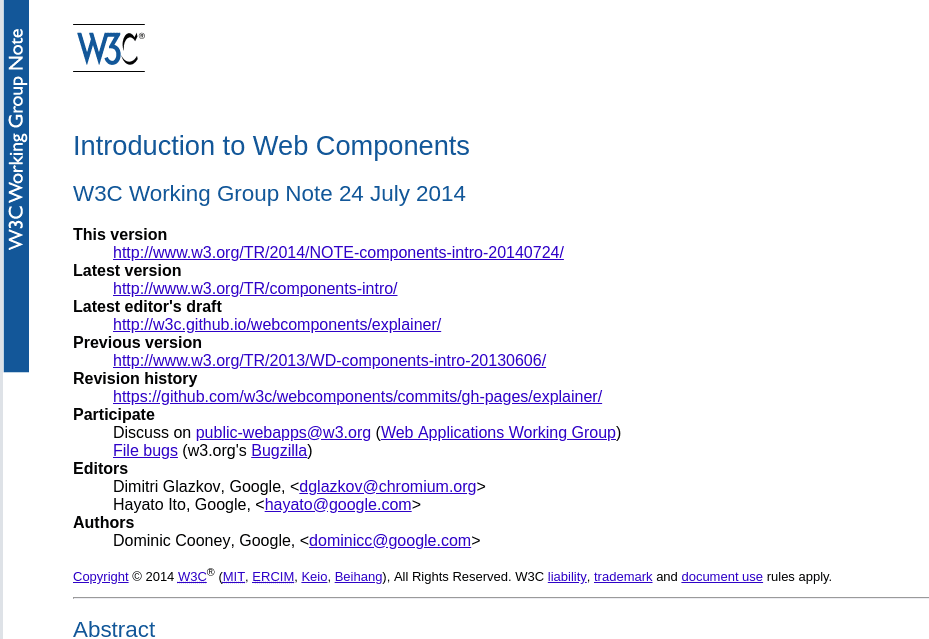
And most major browsers support this new standard:
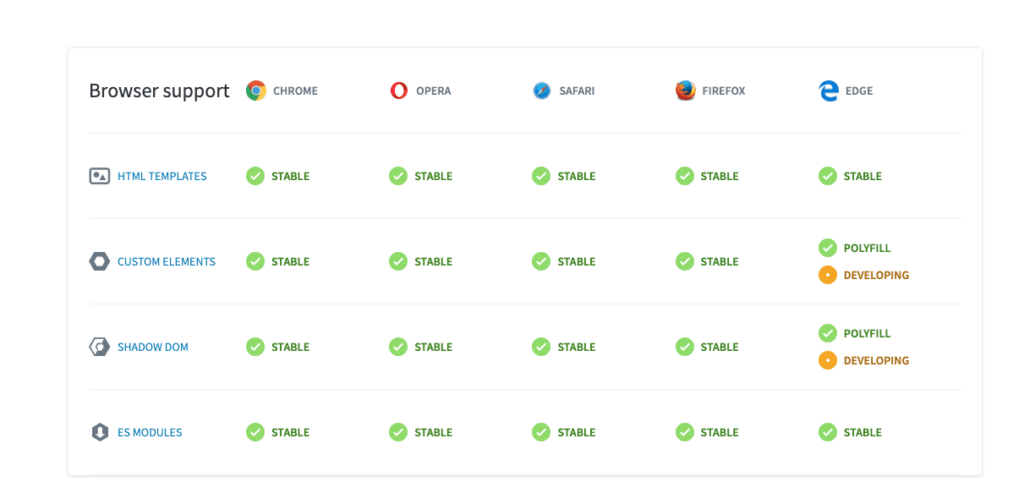
Internet Explorer supports these features using polyfills.
By creating a web component, you are creating a new native DOM element. The situation is not like in React, where a “virtual DOM element” is created and subsequently injected into the DOM.
<my-component hello="world"></my-component>
The nicest aspect of Svelte was that it removes the runtime environment and the same goes for web components. When creating a native DOM element, you do not need a runtime; a regular browser is enough to run the application.
Since these are just DOM elements, any web page can use them. You can create widgets or entire applications, which will then be embedded in existing applications. It doesn’t matter what exactly you program in – Angular, React, Vue or something completely different. These are the most common DOM elements like the <div> tag.
<body>
<my-app name="Hello world"></my-app>
<script src="/my-app.js" type="module"></script>
</body>
This opens up completely new possibilities and new approaches to the architecture of large offerings for the client side. Microfronts are one example of this kind .
It is possible to write web components directly, but this is actually not a good practice from a development standpoint. Better to use a framework that compiles down to the web component level. Two popular frameworks of this kind are Stencil.js and Polymer (from Google).
When using web components, their packages are very compact because there is no runtime. Take a look at the following benchmarks:
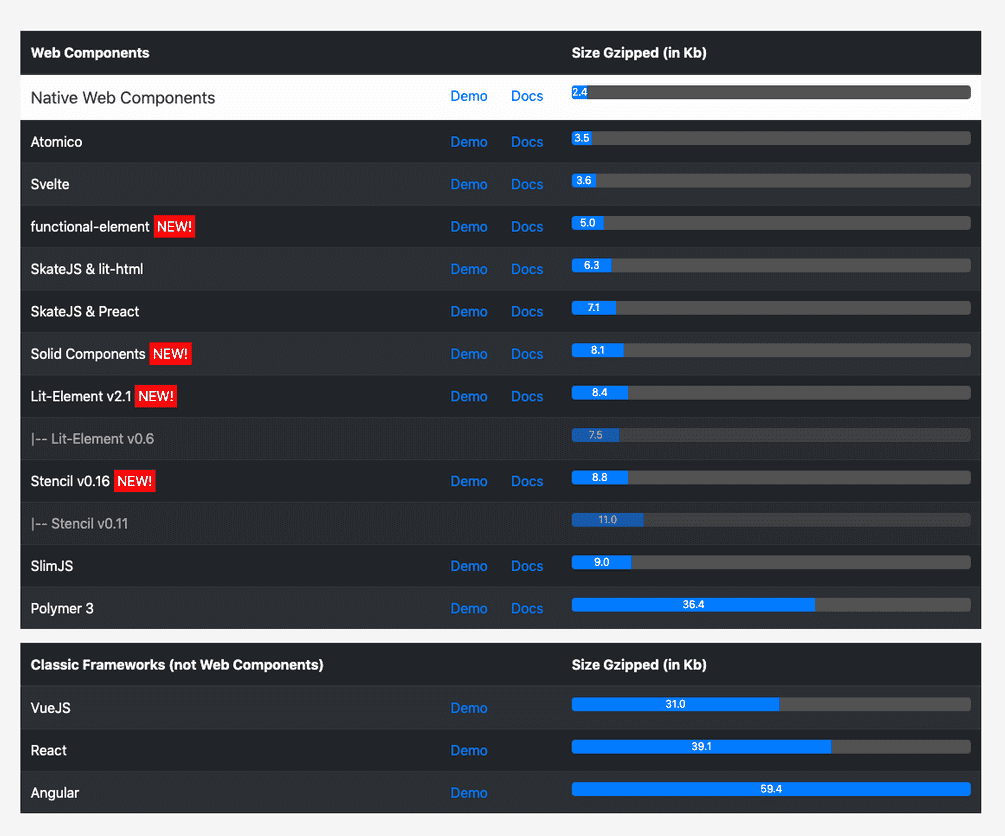
Have you noticed the Svelte mentioned above in this list? Svelte can compile down to the component level, and the package it generates is quite small.
However, it is even more interesting to see how much smaller all the web component framework packages are compared to packages of “classic” frameworks like React and Angular.
How are web components treated in React?
While React can also compile down to web components, it doesn’t support them, according to custom-elements-everywhere.com.

However, full support may follow; perhaps the React team is just going to take this step after thinking it through properly before launching. One of the reasons for this bias is that web components use the imperative style, rather than the declarative style, as in React. Maybe someday React will have full support for web components, or maybe not. In the meantime, if you are going to create web components, use another library instead of React.
Should I use Web Components?
It seems to me that web components are most useful when you use them in a microfront-end architecture. If you work in a large organization with many teams operating at once using different client libraries, then collaborating on code in different teams can be tricky. In this case, the approach to work is different than with React. React specializes in orchestrating an entire application, not creating widgets. As you can see, React and web components can coexist peacefully with each other.
Web Components have roughly the same disadvantages as Svelte. If you’re typing a resume looking to get a job, then web components are not a priority. At least not overriding React. My experience tells me that companies are not as demanding yet (yet?).
Final thoughts
There is a lot of cool and interesting things going on in front-end development. React is just one of the frameworks, and you just need to navigate what is happening outside of it.
If you are a senior developer specializing in frontend, then of course you are looking for the best tools for the job. This is often React, but web components may be a better fit for your next project.
About The Author: Yotec Team
More posts by Yotec Team